Table of Contents
Introduction – Make Python Faster
Python is beloved for its simplicity and versatility, but it’s no secret that it can sometimes be slower than other programming languages. However, don’t let that deter you! With a few tweaks and best practices, you can significantly boost your Python code’s performance. Here are 10 ways to make Python faster, complete with real-life examples to help you grasp these concepts better.
1. Use Built-In Functions and Libraries
Python’s built-in functions and standard libraries are highly optimized and can significantly speed up your code. For example, when you need to calculate the sum of a list, use the built-in sum()
function instead of writing your own loop.
Example:
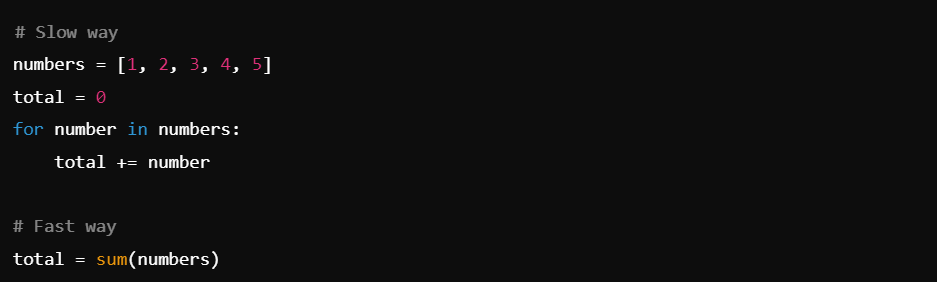
Using the built-in sum()
function is not only faster but also makes your code cleaner and more readable.
2. Use List Comprehensions
List comprehensions are a more Pythonic and faster way to create lists compared to traditional for-loops. They are optimized for performance and can make your code run faster.
Example:
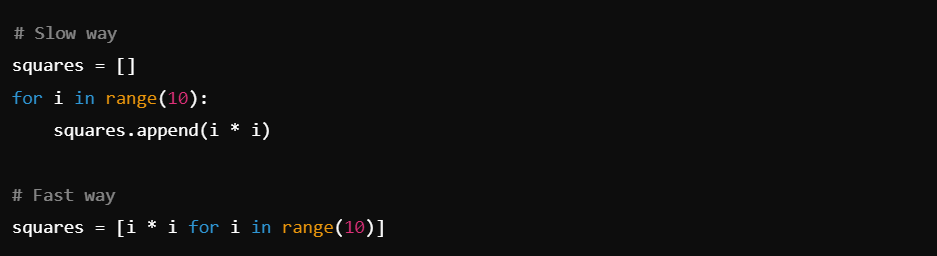
List comprehensions are often more concise and can significantly speed up your code, especially when dealing with large datasets.
3. Avoid Global Variables
Accessing global variables is slower than accessing local variables. Whenever possible, avoid using global variables and pass parameters to functions instead.
Example:
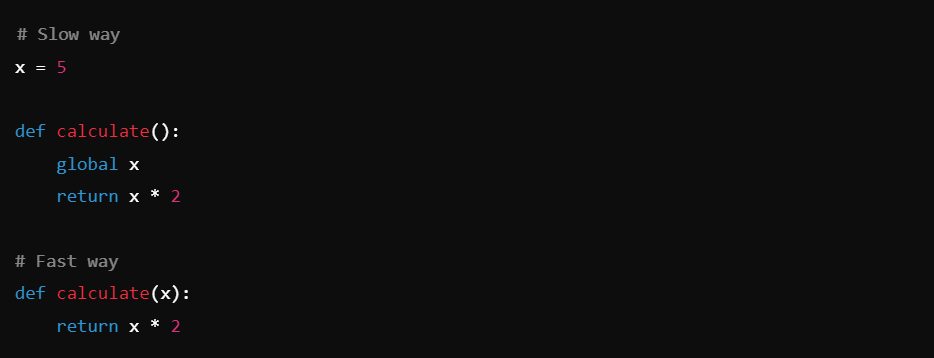
By passing x
as a parameter, you can avoid the overhead of accessing a global variable.
4. Use Generators Instead of Lists
Generators allow you to iterate through data without loading the entire dataset into memory at once. This can save both time and memory, especially with large datasets.
Example:

Generators yield items one by one, which makes them more memory-efficient and faster for large data processing.
5. Profile Your Code
Before optimizing, it’s crucial to identify the bottlenecks in your code. Use profiling tools like cProfile
to find which parts of your code are slow and need optimization.
Example:
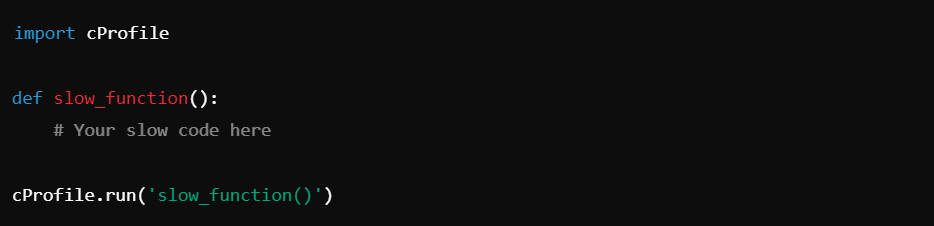
Profiling helps you focus your optimization efforts where they are most needed, saving you time and effort.
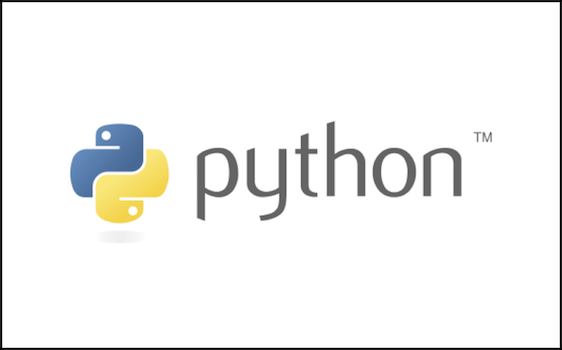
6. Use NumPy for Numerical Operations
NumPy is a powerful library for numerical operations in Python. It can perform large array computations much faster than native Python due to its optimized C and Fortran libraries.
Example:
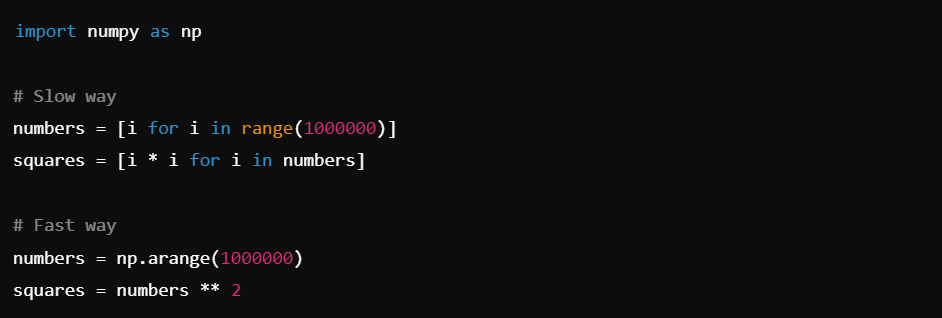
Using NumPy can significantly speed up your numerical computations.
7. Use Multi-threading and Multi-processing
For CPU-bound tasks, multi-threading and multi-processing can help you take full advantage of your CPU cores, thereby speeding up your code execution.
Example:
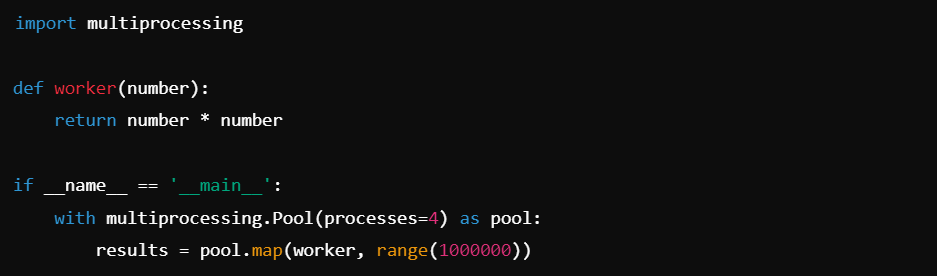
Using multi-processing allows you to parallelize your tasks and reduce execution time.
8. Optimize Loops
Loops are often a major bottleneck in Python code. Minimize the use of loops and try to use vectorized operations or built-in functions whenever possible.
Example:
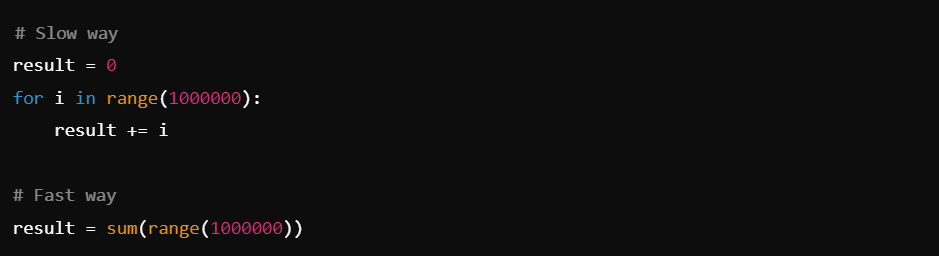
Using built-in functions and avoiding unnecessary loops can drastically improve performance.
9. Use Cython or PyPy
Cython and PyPy are alternative implementations of Python that can offer significant speed improvements. Cython allows you to compile Python code into C, while PyPy is a just-in-time compiler for Python.
Example with Cython:
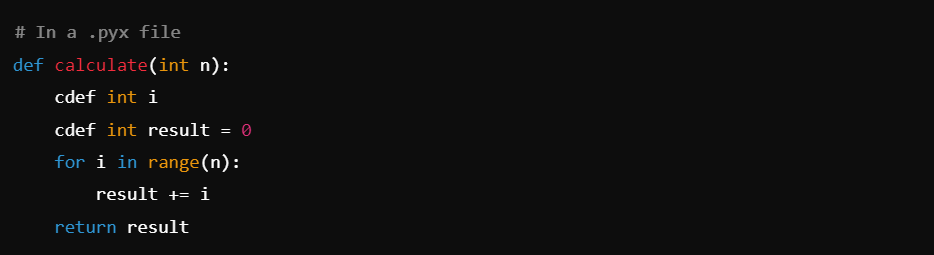
Compiling your code with Cython can lead to major performance gains, especially for computation-heavy tasks.
10. Avoid Unnecessary Abstractions
While Python’s dynamic typing and object-oriented features are powerful, they can introduce unnecessary overhead. Avoid overusing abstractions like classes and inheritance if they are not needed.
Example:
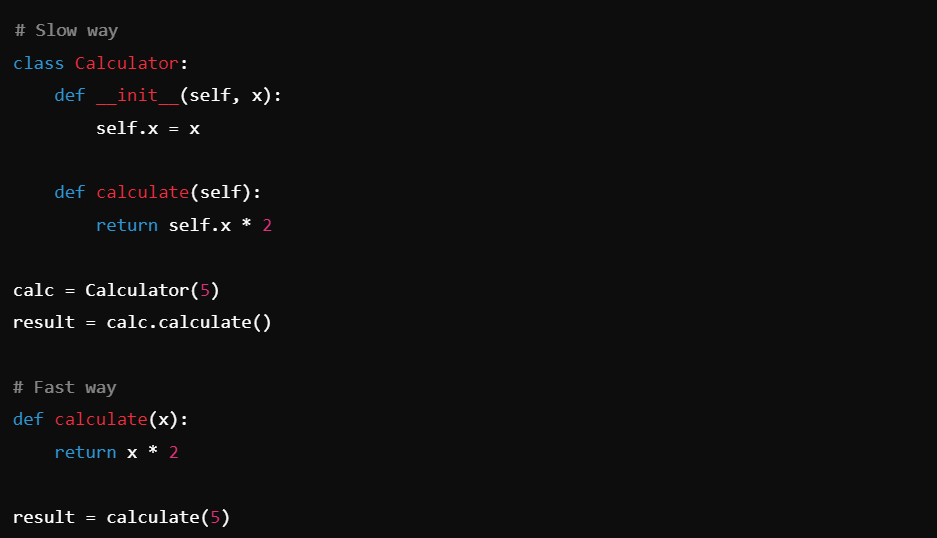
By simplifying your code and avoiding unnecessary abstractions, you can achieve better performance.
Conclusion – Make Python Faster
Making Python faster is all about writing efficient, clean code and leveraging the right tools and techniques. From using built-in functions and libraries to optimizing loops and employing multi-threading, there are numerous ways to enhance the performance of your Python programs. By following these tips and tricks, you can make your Python code run faster and more efficiently, helping you tackle even the most demanding tasks with ease.
So, go ahead and start optimizing your Python code today! Happy coding!
1 Pingback